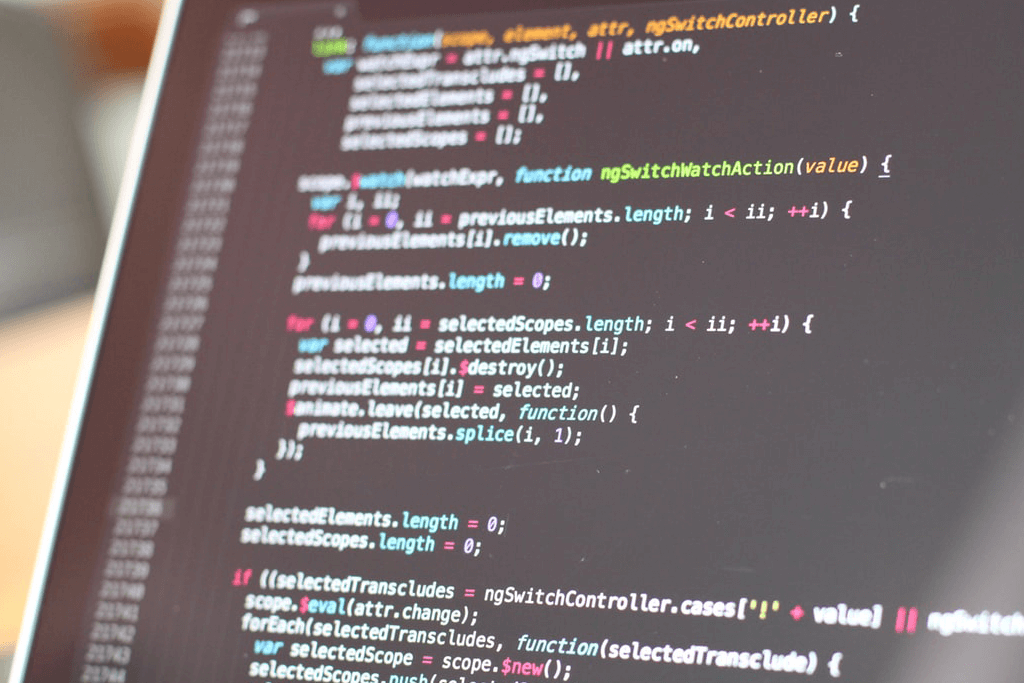
What’s New in Xcode 9
Xcode is the complete developer toolset used to create apps for Apple TV, Apple Watch, iPad, iPhone, and Mac. The Xcode development environment bundles the Instruments analysis tool, Simulator, and the OS frameworks in the form of tvOS SDKs, watchOS SDKs, iOS SDKs, and macOS SDKs.
Highlights of Xcode 9
Xcode 9 includes overall quality improvements as well as extensive new features.
- All new editor.Fast, structure-based editor that lets you intelligently highlight and navigate your code. Includes great Markdown support.
- Refactoring.Refactoring built right into the editing experience and works across Swift, Objective-C, Interface Builder, and many other file types.
- Super-fast search.The Find navigator returns results instantly.
- Debugging.Wirelessly debug iOS and tvOS devices over the network, new debuggers for Metal, and more features throughout Xcode.
- Source Control.All new source control navigator and integrated support for GitHub accounts for quickly browsing repositories and pushing your repositories to the cloud.
- Xcode Server built-in.Continuous integration bots can be run on any Mac with Xcode 9, no need to install macOS Server.
- New Playground templates.Includes iOS templates designed to run well in both Xcode and Swift Playgrounds in iPad.
- New Build System.An opt-in preview of Xcode’s new build system provides improved reliability and performance.
Building and Linking
- New in Xcode 9 – New build system.
- Added a preview of a new build system written in Swift.
- Provides higher reliability.
- Catches many project configuration problems.
- Improves overall build-system performance.Note, build system performance does not include the compilers, linkers, and other tools used by the build system.
- Currently optional and will become the default in a future version of Xcode.
- To opt into the new build system for a project or workspace, choose File > Project Settings or File > Workspace Settings, and then choose New Build System (Preview) for the Build System type.
- For notes on compatibility with existing projects, see the Xcode Release Notes.
Core ML
- New in Xcode 9 – Core ML editor.
- Generate strongly typed interfaces for the model.
- Model compilation for on-device usage.
Debugging
- New in Xcode 9 – Network debugging for iOS and tvOS devices.
- Debug iOS and tvOS devices over WiFi or wired networks.
- Connect via Bonjour or enter an IP address.
- iOS devices need to be plugged-in once to enable network debugging.
- New in Xcode 9 – GPU frame capture and GPU overrides.
- View command buffers, attachments, resources, and call stacks.
- Modify shaders and save changes to your app.
- Move through the timeline for the sequence.
- Inspect and modify values for individual pixels in attachments including color values, alpha, depth, and more.
- Experiment with the rendering state without modifying code by minimizing texture bandwidth, minimizing number of rendered pixels, disabling the blending stage, showing only the wireframe, and scaling the amount of tessellation.
- New in Xcode 9 – Undefined Behavior Sanitizer.
- Use theUndefined Behavior Sanitizerto find sources of program crashes, unexpected behaviors, and incompatibilities with future versions of Xcode.
- Detect several types of undefined behaviors in C languages such as integer overflow, invalid casts, and alignment violations.
- Enable the Undefined Behavior Sanitizer in the Diagnostics pane of the scheme editor.
- New in Xcode 9 – Main Thread Checker.
- Detects AppKit, UIKit, and WebKit method calls that are not made on the main thread.
- Automatically enabled during debugging, and can be disabled in the Diagnostic tab of the scheme editor.
- Main Thread Checkerworks with Swift and C languages.
- Enhanced the Breakpoint navigator with deep filtering.
- Added visual indicators for modified breakpoints.
- Extended the view debugger to show view controllers, and to work with SceneKit and SpriteKit views.
- Enhanced iOS energy gauge.
Metal 2 Support
- New in Xcode 9 – Capture API.
- Define explicit capture boundaries in your code.
- Use the new
MTLCaptureManager
class and the newMTLCaptureScope
protocol to set, manage, and trigger capture boundaries programatically. - Use the extended Xcode GPU Capture UI to start and stop captures manually. You can also use Xcode to capture API boundaries defined in your app.
- New in Xcode 9 – GPU Counters.
- Analyze detailed profiling metrics about a specific GPU capture. In iOS and tvOS, GPU counters are shown as a timeline of command encoders; in macOS, GPU counters are shown as a timeline of draw or dispatch calls.
- Examine runtime performance of render or compute pipeline stages, such as the vertex shader, fragment shader, compute kernel, and more.
- Compare the amount of GPU time spent in each pipeline stage to find your performance bottlenecks.
- New in Xcode 9 – GPU Remarks.
- Find optimization opportunities in your Metal shading language code.
- Debug device-specific runtime issues in the shader editor.
- Follow direct resolutions and instructions to improve your code.
- New in Xcode 9 – Smart Filtering.
- Find specific debug information by typing into the debug navigator. Xcode dynamically displays suggestions as you type and highlight matching text, such as resources, pixel formats, function names, object labels, and more.
- Filter search results by selecting options from a pre-defined menu of Metal objects.
- Use multiple filters with many matching conditions.
- New in Xcode 9 – VR Support.
- View VR submissions and left-eye/right-eye submitted surfaces.
- Added texture inspection for inspecting values of individual pixels in render targets, such as color, alpha, depth, and more.
- Added inspecting output vertex attributes to the buffer editor.
- Added data tips support for Metal objects such as textures, buffers and samplers.
Refactoring
- Global rename across Swift, C, Objective-C, and C++ files.
- View all the changes in one place.
- Converts method signatures between Swift and Objective-C formats.
- Updates properties, getters, setters, and synthesized iVars as needed.
- One button change.
- Fix-it automatically fills in missing cases in switch statements, and mandatory methods for protocol conformance with one click.
- Extract method functionality for all supported languages, along with other language-specific local refactoring.
Simulator
- New in Xcode 9 – Multiple concurrent simulators.
- Run multiple simulators at the same time.
- Run more tests in parallel.
- Test synching and other multi-device workflows.
- New chrome for iOS and watchOS simulators includes the hardware controls, and allow easy dragging and resizing of the simulated device.
- Share information with Simulator from Maps, Photos, and Safari.
- Added option to keep simulators running after closing the window or quitting Simulator for better integration with the
simctl
command of thexcrun
command line tool. - Record videos of simulators.
- Get help by choosing Help > Simulator Help.
Source Control
- New source control navigator for viewing branches, tags, and remote repositories for the current workspace.
- New source control inspector shows details for the selected navigator item.
- New editor for branch history including a jump bar for easy navigation.
- New side-by-side editor for file diffs.
- Easier and faster access to common tasks.
- GitHub account integration for easy browsing and one-click creation of a project and the associated GitHub repository.
Source Editing
- New in Xcode 9 – All new source editor.
- Faster and more versatile Find and replace.
- Fast scrolling for any sized file.
- Direct manipulation of code structure such as tokens and blocks.
- Redesigned integration for source control.
- Redesigned presentation of error and warning messages.
- Support for Markdown.
Swift
- New in Xcode 9 – Swift 4.
- One compiler for Swift 4 and Swift 3, Swift 4 and Swift 3 targets can be compiled together in the same project.
- Improved migrator experience that supports migrating only select targets to Swift 4.
- Faster generic code and decreased code size.
Testing
- New in Xcode 9 – Parallel device testing.
- Added new APIs to XCTest.
- Control and capture screenshots.
- Group test activities.
- Test attachments.
- Cleanup test state in a teardown block.
- Target multiple apps in one UI test.
- Run tests using a specified language and region.
Xcode Server
- New in Xcode 9 – Built in Xcode Server.
- New Server & Bots pane in Preferences for configuring Xcode Server and for setting Bot permissions.
- Added support for 2-factor authentication.
- Updated functionality for bots.
- Support automatic and manual provisioning workflows.
- Pass additional arguments to
xcodebuild
. - Run tests in parallel on devices and Simulator.
- Configure language and region for tests.
- Send “all clear” emails notifications.
Compatibility
Xcode 9.0 requires a Mac running macOS Sierra 10.12.4 or later.
Installation
Xcode 9 beta can coexist with previous versions of Xcode.
Prerelease versions of Xcode are made available fromdeveloper.apple.comto authorized seed developers, packaged in a compressed XIP file. To install Xcode during the beta period, download the XIP file, drag it to the Applications folder, then double-click the file. The XIP file expands in place.
Note:If you have enabled “Open ‘safe’ files after downloading” in Safari Preferences, the XIP file downloads and is automatically expanded in your download file folder. Drag Xcode-beta to the Applications folder before launching it.
Upon final release, Xcode is installed via the Mac App Store.
Good luck!