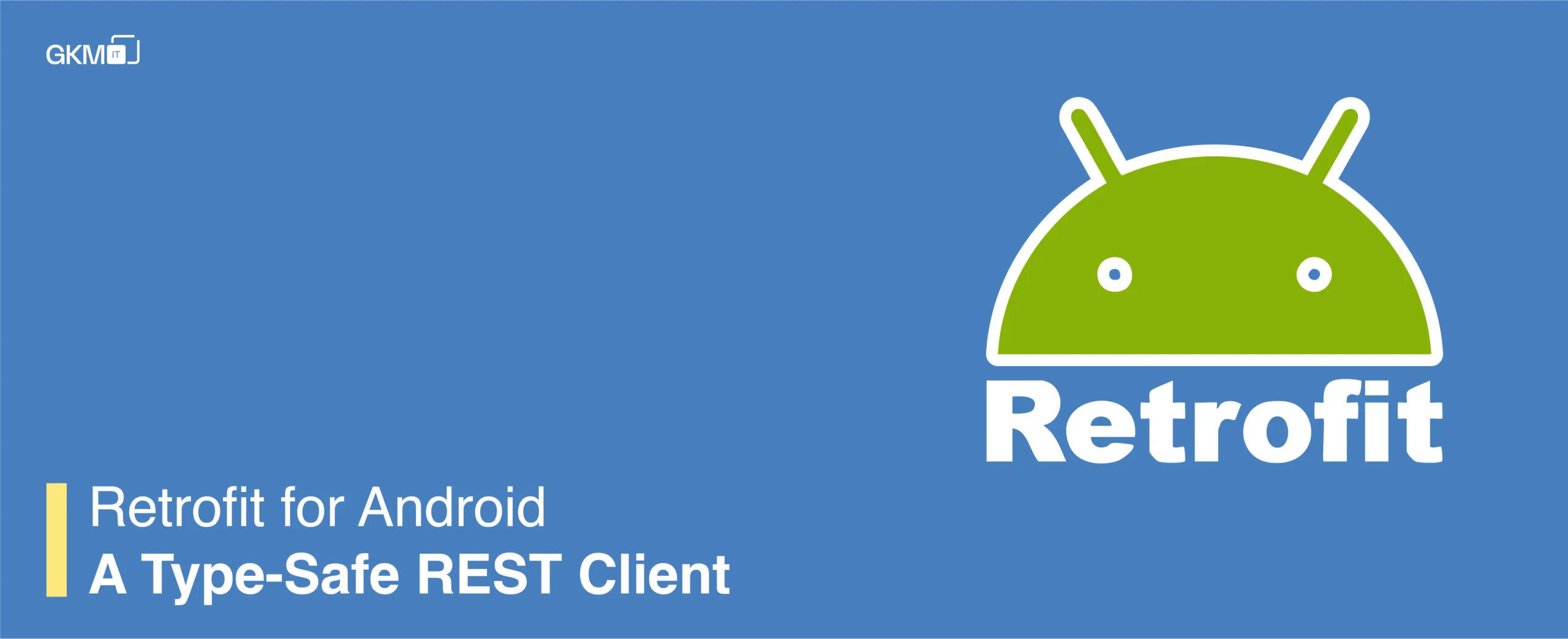
Retrofit Android, A type-safe Rest client
What is Retrofit?
Retrofit is a REST Client for Android, By using Retrofit in Android we can easily retrieve and upload JSON ( or other structured data like RSS feed, etc. ) responses from restful web APIs. It is different from other libraries because Retrofit gives us an easy-to-use platform through which we don’t need to parse JSON responses as they are done by the library itself. It uses the GSON library in the background to parse the responses. All we need to do is define a POJO(Plain Old Java Object) to do all the parsing. Retrofit is widely used in Mobile App Development Services, as it simplifies API integration and enhances network communication efficiency.
Features of Retrofit 2.0
Retrofit 2.0 comes with a lot of features. Some of them are listed below:
-
Declaration for synchronous and asynchronous API calls made same.
-
Retrofit 2 gives a Call<T> object for calling any API. Which can be canceled later on.
-
The converter is now excluded from Retrofit. You have to use converters of your choice separately.
-
It makes the library very tiny Here is the list of official Converter modules provided by Square.
-
Choose one that fits your requirements best.
- Gson: com.squareup.retrofit:converter-gson
- Jackson: com.squareup.retrofit:converter-jackson
- Moshi: com.squareup.retrofit:converter-moshi
- Protobuf: com.squareup.retrofit:converter-protobuf
- Wire: com.squareup.retrofit:converter-wire
- Simple XML: com.squareup.retrofit:converter-simple xml
Getting Started with Retrofit 2.0
For basic understanding retrofit three classes:
-
POGO Classes to map structured data.
-
Interface class to define HTTP calls with methods.
-
Retrofit.Builder class.
1 . POGO class: The Simple model classes have properties, getters, and setters for respective properties.
Below is the sample class for that:
2. Interface Class:
In this class methods for each Api Call define with HTTP annotation (GET, POST, etc.) to specify the request type and the relative URL,.The return value wraps the response in a Call object with the type of the expected result.
First of all, we have to specify the method type we want to use for API calls.
@GET , @POST , @PUT, @DELETE, @UPDATE etc.
Then we will specify Call<T> where T is the response class as the return type of method.
If API requires the multipart request or FormData request then we have to specify @Multipart, @FormUrlEncoded.
In GET request or POST with a JSON request does not need extra annotations
For POST(FormData) requests we have to specify the request as the @Field parameter.
For the POST(Multipart) request, we have specified the request as @Part.
For POST(JSON) requests we have to specify the request as @Body with Request Class.
For the @GET request, we can specify @Query or @Path Parameter.
Below are the sample methods for different types of requests:
3. Builder Class:
Retrofit builder allows headers using Authentication with OkHttp interceptors, base URL converter factory, etc . for the HTTP calls.
That’s it now u just need to Asynchronous Call to the server:
You can download the full source from the following Github link. If you Like this tutorial, Please star it on Github.