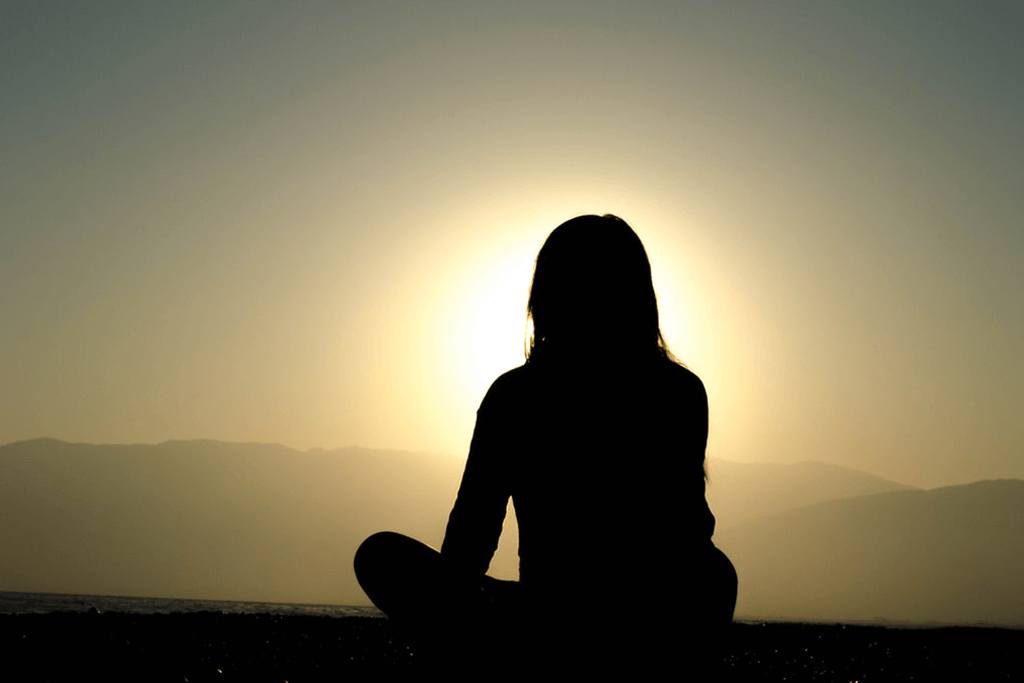
OAuth 1.0a “one legged” authentication with Ruby on Rails
OAuth 1.0a is an old method of authentication but is still in use by a lot of legacy third parties as a layer of authentication for their APIs.
OAuth 1.0a provides five modes –
- One Legged
- Two Legged
- Three Legged
- Echo
- xAuth
To learn more about different modes and OAuth refer to their official bible – http://oauthbible.com/
This article talks about how to perform one legged authentication with Ruby on Rails.
The basic mechanism is as shown in the image below –
Ref – http://oauthbible.com/
There are only two things that you require for performing an OAuth 1.0a authentication –
- Consumer Key
- Consumer Secret
The gem that works well for one legged approach is https://github.com/oauth-xx/oauth-rubyThere are other gems that can be used but I found this to be pretty simple and straightforward.
Steps to follow –
1. Install the OAuth gem
sudo gem install oauth
or add it to your gemfile and perform bundle install
gem 'oauth'
2. Create a consumer to consume various API endpoints
consumer=OAuth::Consumer.new('consumer_key', 'consumer_secret', :site => 'https://example.com')
3. How to make a GET call?
response = consumer.request(:get, ‘/resource/:id’, nil, {}, {})
puts response
4. How to make a POST call?
params = { "key1": "value1", "key2": "value2" }
response = @consumer.request(:post, '/resource', nil, {}, params)
If you need to specify Content-Type
response = @consumer.request(:post, '/resource', nil, {}, params,
{ 'Content-Type' => 'application/json' })
puts response
5. How to make a PUT call?
params = { "key1": "value1", "key2": "value2" }
response = @consumer.request(:put, '/resource', nil, {}, params.to_json)
If you need to specify Content-Type
response = @consumer.request(:put, '/resource', nil, {}, params.to_json,
{ 'Content-Type' => 'application/json' })
puts response
Please note that for the PUT method the params have to be strictly converted to JSON.
In case you want to test the OAuth 1.0a one legged authentication over postman, refer to the screenshot below
I hope the above steps helped you to clear all your doubts. Still, If you have any queries or want to understand it in a better way. Please leave your comments and feedback in the comment section below. I will be glad to assist you.