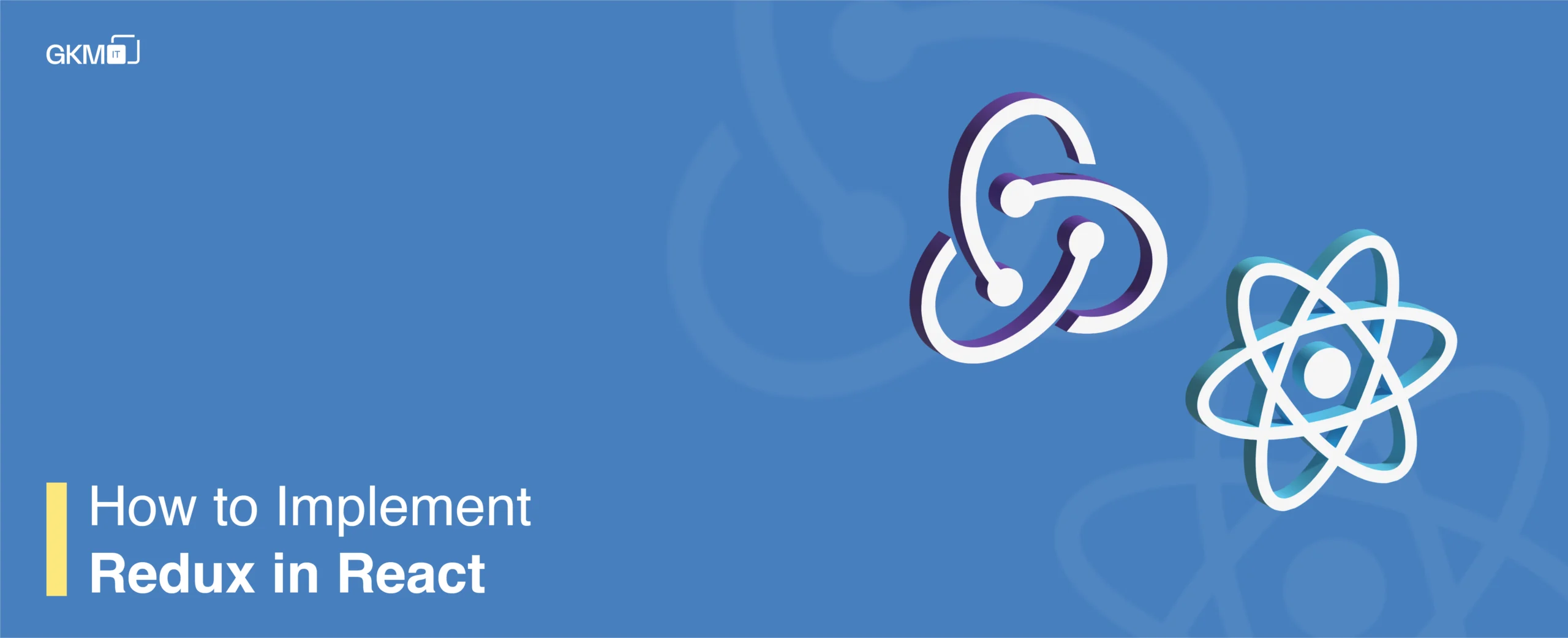
How to Implement Redux in React
What is Redux?
Redux is a predictable state container for JavaScript apps. It has a single Store and cannot have multiple Stores. It divides the Store into various state objects, making state management easier and more structured.
Importance of State Management in Frontend Development
Efficient state management is crucial for seamless user experiences in modern web applications. Technologies like Redux help handle complex state changes efficiently, making them a valuable part of Frontend Development Services.
What is React?
1) React isย a Javascript library created byย Facebook
2) React is a User Interface library
3) React is a tool for building UI components
What is a Store?
A store holds the whole state tree of your application.
A store has the following responsibilities:
- Holds the entire application state.
- Allows access to the state via
getState()
. - Enables state updates through
dispatch(action)
.
What are Reducers?
Reducers specify how the application’s state changes in response to actions sent to the store. They take the previous state and an action, then return a new state based on the action type.
What are Actions?
Actions are payloads of information that send data from your application to your store. They are the only source of information for the store. Actions ensure the unidirectional data flow, a core principle of Redux.
Installation of Redux with React-
Installation includes three steps and to make it easy to understand I have explained below through codes/commands which I have worked on while working on my project. I have created three files index.js, app.js, and reducer.js to explain it simply. To understand in deep please read the commands I have worked on.
commands-
npm install -g create-react-appcreate-react-app react-with-redux-implementnpm install --save redux react-redux
Below given code is an example to show how redux is implemented with React.
Index.js
import React from 'react';import ReactDOM from 'react-dom';import './index.css';import App from './App';import * as serviceWorker from './serviceWorker';import { createStore } from 'redux';import { Provider } from 'react-redux';import appState from './reducers/reducer'; const store = createStore(appState, window.__REDUX_DEVTOOLS_EXTENSION__ && window.__REDUX_DEVTOOLS_EXTENSION__());ReactDOM.render( <Provider store={store}> <App/> </Provider>, document.getElementById('root'));serviceWorker.unregister();
App.js
import React from 'react';import './App.css';import { connect } from 'react-redux'; function App(props) { return ( <div className="app" style={{textAlign:"center"}}> <h1>React and Redux Example</h1> <h3>This Value is Reducer State Value: <span style={{color:"gray"}}>{props.name}</span></h3> <button onClick={ ()=>{ props.hanldeChange("Era") }}>Change It</button> </div> );} const mapStateToProps = (state) => { return { name: state.name }} const mapDispatchToPorps = (dispatch) => { return { hanldeChange:(name) => { dispatch({type:"CHANGE_NAME", payload: name}) }}} export default connect(mapStateToProps, mapDispatchToPorps)(App);
Reducer js
const isState = { name: "Rock", wishes: ["code", "game"]} const reducer = (state=isState, action) => { if(action.type === 'CHANGE_NAME'){ return { ...state, name: action.payload } } return state;} export default reducer;
Why Use Redux with React?
Using Redux in React applications offers several benefits:
- Centralized State Management: Keeps all application data in a single store.
- Predictability: Ensures consistent behavior across different states.
- Improved Debugging: With tools like Redux DevTools, you can track state changes easily.
- Scalability: Makes handling complex applications more manageable.
Conclusion
Through the above codes and examples, I have explained how to implement and connect Redux with React in a simple way. This guide will help you build scalable applications with efficient state management. If you have any questions, feel free to ask in the comment section below!